In the world of software development, managing changes to your code base is crucial. One of the most powerful tools at your disposal for handling these changes is Git. However, there are times when you might need to undo a commit due to mistakes or changes in direction. This guide will walk you through the process of uncommitting in Git step-by-step, ensuring that you have a solid understanding of the concepts and commands involved.
Understanding the Basics of Git
Before diving into uncommitting, it's essential to grasp some foundational concepts of Git. Understanding how Git operates will make the uncommitting process smoother and more intuitive.

What is Git?
Git is a distributed version control system that allows teams and developers to track changes in source code over time. Unlike centralized version control systems, Git gives each developer a local copy of the entire repository, which enhances collaboration, branching, and revision management.
With Git, every change made is documented through commits, which are snapshots of your project’s history. This robust system enables you to manage various versions of your project effectively, making it easier to revert to earlier states when necessary. The ability to create branches for new features or experiments means that developers can work in isolation without affecting the main codebase, thereby reducing the risk of introducing bugs into the production environment.
Importance of Version Control in Coding
Version control is a crucial aspect of modern software development. It provides a safety net for developers, allowing them to collaborate on shared projects without fear of losing work or overwriting each other's changes.
Using version control systems like Git helps in maintaining project integrity, fostering collaboration, and tracking project history. It allows teams to work simultaneously on different features while providing the ability to merge changes seamlessly. Furthermore, version control systems facilitate code reviews, enabling team members to provide feedback on each other's work before it is integrated into the main project. This collaborative review process not only improves code quality but also enhances team communication and knowledge sharing, as developers become more familiar with each other’s contributions and coding styles.
Additionally, Git's branching capabilities allow for experimentation without the risk of destabilizing the main project. Developers can create branches for new features, bug fixes, or even exploratory work, and once the changes are verified, they can be merged back into the main branch. This practice promotes a culture of innovation, where developers feel empowered to try new ideas without the fear of permanent consequences. As a result, teams can iterate quickly and efficiently, adapting to changing requirements and improving their overall productivity.
The Concept of Uncommitting in Git
Uncommitting in Git refers to the action of removing or editing a commit. This might be necessary if a mistake was made in the code or if the commit needs to be altered for any reason.
What Does Uncommit Mean?
To "uncommit" essentially means to undo the last commit you made. It can involve resetting the commit entirely or creating a new commit that reverses the changes of the previous one. Understanding the difference between these options is key to mastering Git.
Why Would You Need to Uncommit?
There are several scenarios that might prompt you to uncommit in Git:
- Incorrect code: If the latest commit contains errors or bugs that need to be addressed.
- Improper commit message: Sometimes, a commit may have a misleading or incorrect message that needs to be corrected.
- Unfinished code: If you've submitted code that isn't fully functional or complete, uncommitting allows you to refine it before pushing to a shared repository.
In addition to these scenarios, uncommitting can also be useful when collaborating with a team. If you realize that your recent changes conflict with others' work or if they don't align with the project's direction, uncommitting allows you to reassess your contributions. This can help maintain a clean and coherent project history, which is crucial in collaborative environments where multiple developers are working on the same codebase.
Moreover, uncommitting can serve as a learning opportunity. By revisiting your previous commits, you can better understand your coding habits and identify patterns in your mistakes. This reflective practice not only improves your coding skills but also enhances your ability to write clearer commit messages and structure your commits more effectively in the future. Embracing the uncommit process can lead to a more disciplined and thoughtful approach to version control.
Precautions Before Uncommitting
Before you start the process of uncommitting, there are several considerations and precautions to keep in mind to avoid data loss or messy situations in your repository.
The Risks of Uncommitting
Uncommitting can lead to issues if not done correctly. Some potential risks include:
- Data loss: You may unintentionally lose changes if you reset without careful consideration.
- Conflicts in collaborative environments: Uncommitting shared commits can create discrepancies in team members’ repositories.
It's crucial to be aware of these risks before proceeding.
Safe Practices Before Uncommitting
To ensure a safe uncommitting process, consider the following best practices:
- Create backups: Consider creating a new branch or tagging the commit to preserve changes before proceeding.
- Communicate with your team: If you're working in a team, make sure to coordinate with your team members to prevent confusion.
- Review commits: Familiarize yourself with the changes made in the commit you wish to uncommit.
Additionally, it's wise to utilize version control tools that allow you to visualize the commit history. Tools like Git GUI clients can provide a more intuitive understanding of your repository's state, making it easier to identify the exact changes you want to revert. This visual representation can help you avoid mistakes that might occur when relying solely on command-line instructions.
Furthermore, consider running tests after uncommitting, especially if the changes were significant. Automated testing can catch potential issues early, ensuring that your codebase remains stable and functional. By integrating these practices into your workflow, you can significantly reduce the risks associated with uncommitting and maintain a healthy development environment.
Step-by-Step Guide to Uncommit in Git
Now that you have a grasp on the necessary precautions, let’s dive into the step-by-step process of uncommitting in Git.

Checking Your Commit History
Before uncommitting, it’s vital to check your commit history to confirm which commit you want to remove or alter. Use the following command:
git log
This command will display a list of the most recent commits, along with their hashes. Identify the commit you want to uncommit and take note of its hash for later use. Understanding the context of your commit history can also be beneficial; it allows you to see the evolution of your project and the specific changes made over time. You can even use additional flags with `git log`, such as `--oneline` for a more concise view or `--graph` to visualize the branching structure of your commits.
Using the Git Revert Command
If you want to undo the changes of a particular commit while preserving the commit history, the `git revert` command is your best option. This command creates a new commit that reverses the changes made in the specified commit.
To use `git revert`, execute the following command:
git revert [commit_hash]
This will launch your default text editor, allowing you to modify the commit message for the new commit that will reverse the changes. It’s important to note that `git revert` is particularly useful in collaborative environments where maintaining a clear and accurate commit history is crucial. By creating a new commit instead of deleting the old one, you ensure that all team members can see what changes were made and why they were undone, which can be invaluable for debugging and understanding project decisions.
Using the Git Reset Command
If you prefer to remove the commit entirely and don’t mind losing the changes, the `git reset` command is appropriate. You can use it in two primary modes: soft and hard.
- Soft Reset: This keeps your changes in the staging area while removing the last commit.
Execute:
git reset --soft HEAD~1
Execute:
git reset --hard HEAD~1
Be cautious with a hard reset, as it cannot be undone easily. It’s advisable to use this command only when you are certain that the changes in the commit are no longer needed and you have backups or a reliable way to recover your work if necessary. Additionally, if you are working in a shared repository, consider the implications of a hard reset on your collaborators, as it can lead to confusion and conflicts in the project’s history.
Troubleshooting Common Issues
As with any powerful tool, using Git can sometimes lead to challenges. Here are some common issues you might encounter while uncommitting.
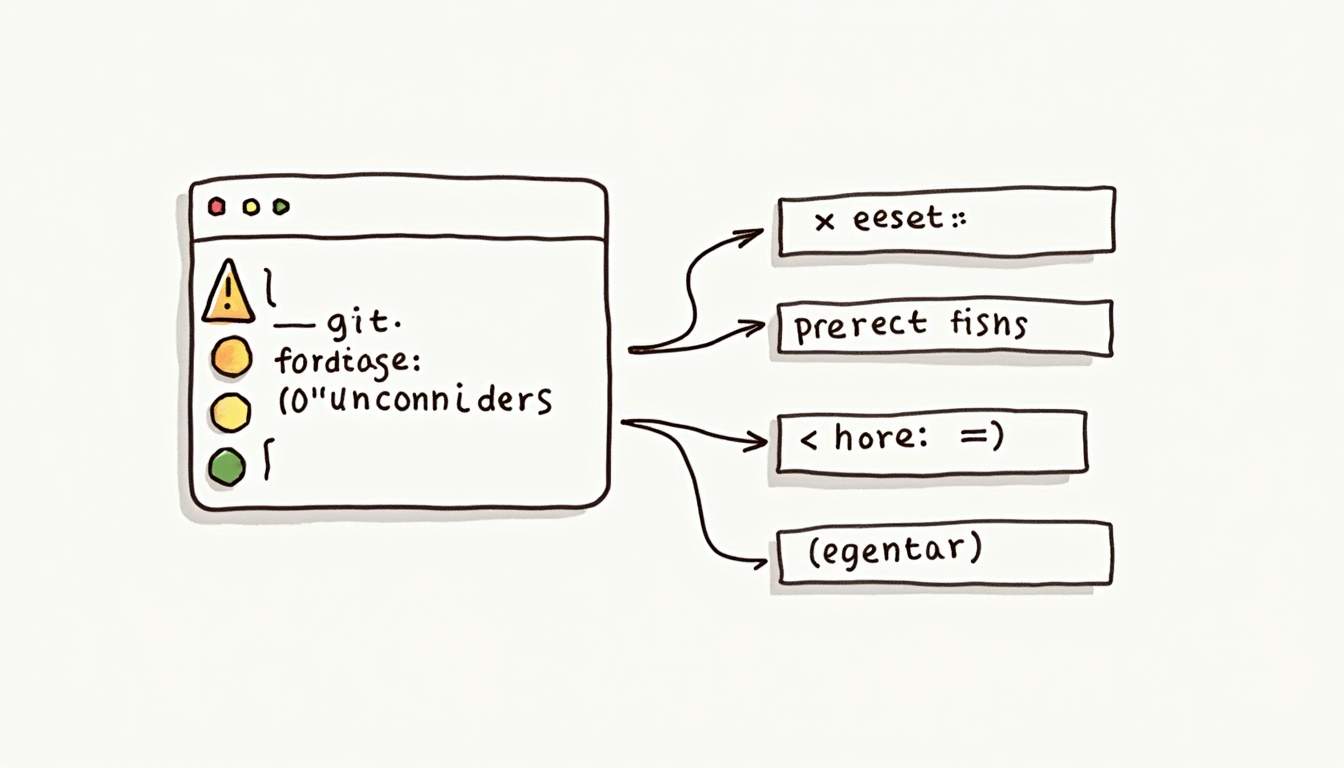
Resolving Merge Conflicts
Sometimes, uncommitting can lead to merge conflicts, especially if you’re working in a shared repository. If this occurs, Git will notify you and provide instructions on resolving the conflicts before you can proceed.
To resolve merge conflicts, you’ll need to manually edit the conflicting files to incorporate changes from both commits, then add the resolved files and commit the changes.
Recovering Lost Commits
If you accidentally reset or reverted and lost important commits, don’t panic. Git has a built-in mechanism to help you recover lost work. Use the following command to view the reflog, which shows a log of where your HEAD has been:
git reflog
This will allow you to find lost commits and restore them as needed.
In conclusion, uncommitting in Git involves understanding its implications, following best practices, and using the right commands. With these steps and precautions, you can confidently manage your commits in Git and enhance your coding workflow.
Streamline Your Development Workflow with Engine Labs
Now that you've mastered the art of uncommitting in Git, take your software development to the next level with Engine Labs. Engine is not just another tool; it's an AI-powered software engineer that integrates with your favorite project management platforms, turning tickets into pull requests with ease. Imagine automating half of your tickets, slashing through backlogs, and shipping projects at a pace you never thought possible. Ready to revolutionize your team's productivity and focus on creating outstanding software? Get Started with Engine Labs today and experience the future of software engineering.