Git is a powerful version control system that allows developers to collaborate and manage codebases effectively. One essential aspect of using Git is the concept of branches. In this guide, we'll walk you through the process of deleting a branch, both locally and remotely. We'll also discuss common errors you may encounter and best practices for managing your branches.
Understanding Git Branches
Git branches are essentially pointers to specific commits in your repository. They allow you to work on different features or fixes simultaneously without interfering with the main codebase. Each branch represents an independent line of development.
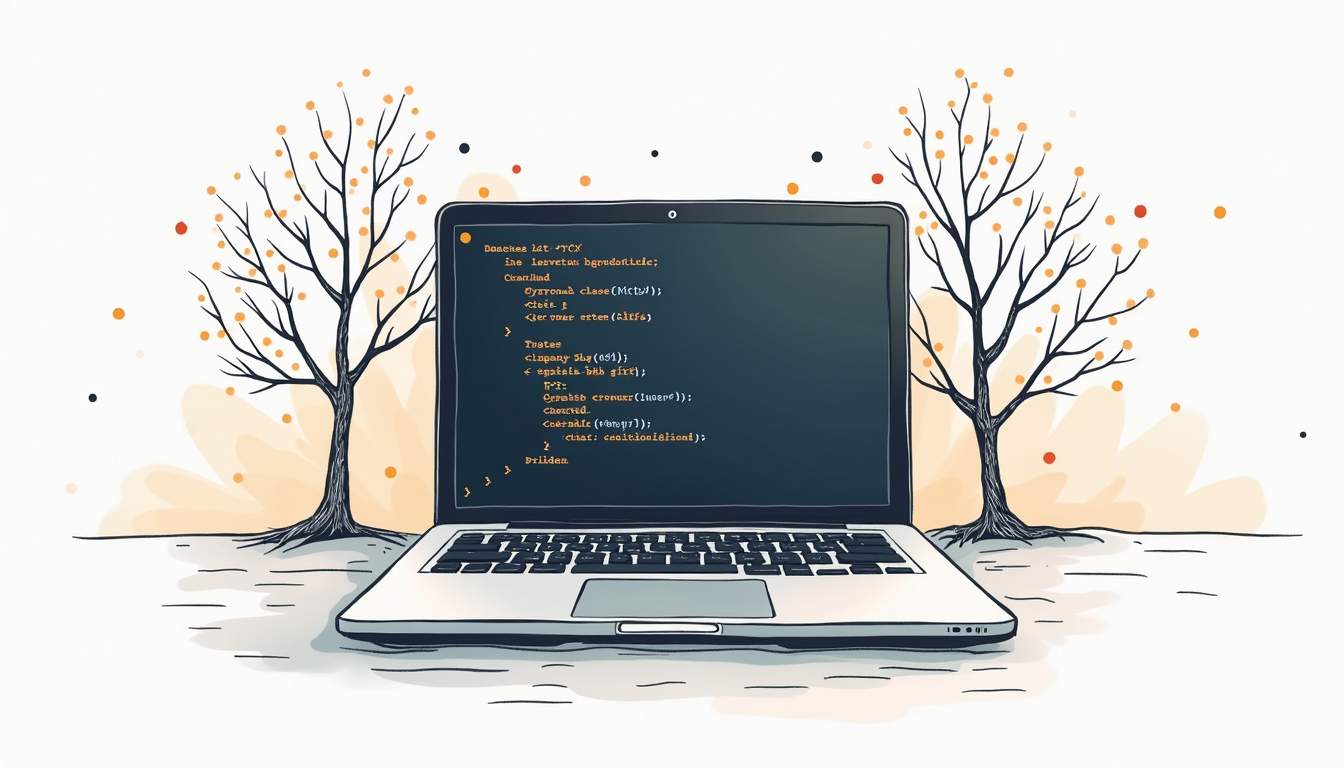
What is a Git Branch?
A Git branch is the fundamental unit of development in Git. When you create a new branch, Git creates a pointer that points to the current commit. This makes it easy to switch between different versions of your code. The default branch in Git is typically called "main," but you can create as many branches as you need. Each branch can have its own unique history, allowing for a clear separation of changes and features. This modular approach not only enhances organization but also simplifies the process of tracking changes over time.
Why Do We Need Git Branches?
Branches facilitate parallel development. They allow multiple developers to work on different features simultaneously without risk of overwriting each other's work. Furthermore, branches help you experiment with new ideas while keeping the main codebase stable. Once a feature is complete, the branch can be merged back into the main line of development. This merging process can involve resolving conflicts if changes in different branches overlap, which is a crucial skill for any developer. Additionally, using branches effectively can lead to a more streamlined workflow, as teams can prioritize tasks and manage releases more efficiently.
Best Practices for Using Git Branches
To maximize the benefits of branching, it's essential to adopt best practices. For instance, naming conventions for branches can greatly enhance clarity; using descriptive names that reflect the feature or bug being addressed helps team members understand the purpose of each branch at a glance. Moreover, regularly merging changes from the main branch into your feature branches can help minimize conflicts and keep your work up to date with the latest developments. It's also advisable to delete branches after they have been merged to maintain a clean repository, preventing clutter and confusion over time.
Common Branching Strategies
There are several branching strategies that teams can adopt to optimize their workflows. The Git Flow model, for example, introduces dedicated branches for features, releases, and hotfixes, providing a structured approach to managing the development lifecycle. Alternatively, the GitHub Flow is a simpler model that focuses on short-lived branches for features and encourages continuous integration. Understanding these strategies can help teams choose the right approach based on their project needs and team dynamics, ultimately leading to more efficient development processes.
Preparing to Delete a Branch in Git
Before you delete a branch, it’s important to prepare accordingly. Deleting a branch that is actively being used can lead to loss of work, so follow these steps to ensure you're ready to proceed.

Checking Out the Branch You Want to Delete
To delete a branch, you must first ensure you are not currently on that branch. You can switch to another branch using the `git checkout` command. For example, if you want to delete a branch named "feature-xyz," switch to the "main" branch with:
git checkout main
By doing this, you can remove the target branch without any conflicts. It’s also a good practice to double-check the branches available in your repository by using the command:
git branch
This command lists all local branches, allowing you to confirm that you are on the correct branch before proceeding with the deletion. Additionally, if you are working in a collaborative environment, it’s beneficial to communicate with your team members about the branch deletion to avoid any surprises or confusion.
Ensuring You Have the Latest Version of Your Branch
Before deleting a branch, it's wise to ensure that you have the latest version of your work and that any changes are either merged or backed up elsewhere. You can do this by running:
git fetch
This command updates your local repository with any changes made to the remote repository, ensuring your branches are up-to-date. Following this, it’s prudent to check the status of your branch with:
git status
This will show you if there are any uncommitted changes or files that need attention. If you find that there are changes that you want to keep, consider creating a patch or a backup branch before deletion. You can create a backup branch using:
git checkout -b backup-feature-xyz
This way, you can safely preserve your work and ensure that nothing is lost during the deletion process. Taking these precautions can save you from potential headaches later on, especially in projects where multiple contributors are involved.
The Process of Deleting a Git Branch
Once you’ve prepared yourself by verifying your current branch and ensuring your changes are stored securely, you can proceed with the deletion process.
Deleting a Local Git Branch
To delete a local branch, use the `git branch` command followed by the `-d` flag and the name of the branch you want to delete:
git branch -d feature-xyz
If the branch has unmerged changes and you’re certain you want to delete it, use the `-D` flag to force the deletion:
git branch -D feature-xyz
This action removes the local reference to the branch, making your repository cleaner and easier to navigate. It’s a good practice to regularly clean up old branches that are no longer in use, as this can help prevent confusion and maintain an organized workflow. Additionally, you can list all your branches using the `git branch` command to identify which branches can be safely deleted.
Deleting a Remote Git Branch
To delete a branch from a remote repository, you will use the `git push` command followed by the `--delete` flag and the name of the branch:
git push origin --delete feature-xyz
This command instructs Git to remove the specified branch from the remote repository. Always ensure that the branch is no longer needed by you or your team before proceeding with this action. Deleting a remote branch can have implications for other collaborators, especially if they are still working on that branch or have not merged their changes. It’s advisable to communicate with your team and confirm that everyone is on the same page regarding the branch's status before executing the deletion.
Furthermore, after deleting a remote branch, it's a good idea to inform your team members to prune their local references to the deleted branch. They can do this by running the command:
git fetch --prune
This command helps to clean up any stale references to branches that no longer exist on the remote, ensuring that everyone’s local repository remains tidy and up-to-date.
Common Errors When Deleting Git Branches
Even experienced Git users may face errors when deleting branches. Understanding common issues can help troubleshoot and resolve problems efficiently.
Dealing with 'Branch not found' Errors
If you encounter an error stating "branch not found," this usually means that the branch name was incorrectly specified or that the branch does not exist in your local or remote repository. Verify the branch name using the command:
git branch
This will list all your local branches and help you confirm the correct name. Additionally, if you're working with remote branches, you can check for their existence with:
git branch -r
This command will show you all remote branches, allowing you to ensure that the branch you're trying to delete actually exists on the remote repository. If you find that the branch does exist remotely but not locally, you may need to fetch the latest updates from your remote repository using:
git fetch
After fetching, you can try deleting the branch again.
Resolving 'Unmerged changes' Errors
If you attempt to delete a branch with unmerged changes, Git will prevent you from deleting it to avoid data loss. In this case, review the changes on the branch and decide if you want to merge or save them elsewhere before proceeding. You can check the status of your changes by using:
git status
This will provide you with a clear overview of any uncommitted changes that may be present. If you decide that the changes are no longer needed, you can forcefully delete the branch using:
git branch -D branch_name
However, exercise caution with this command, as it will permanently remove the branch and its changes without any confirmation. To prevent accidental data loss, consider creating a backup branch before deletion, which can be done with:
git checkout -b backup_branch_name
This way, you can always refer back to the previous state of your work if necessary.
Best Practices for Managing Git Branches
Effectively managing your branches will lead to a more organized project and seamless collaboration. Here are some best practices to follow.
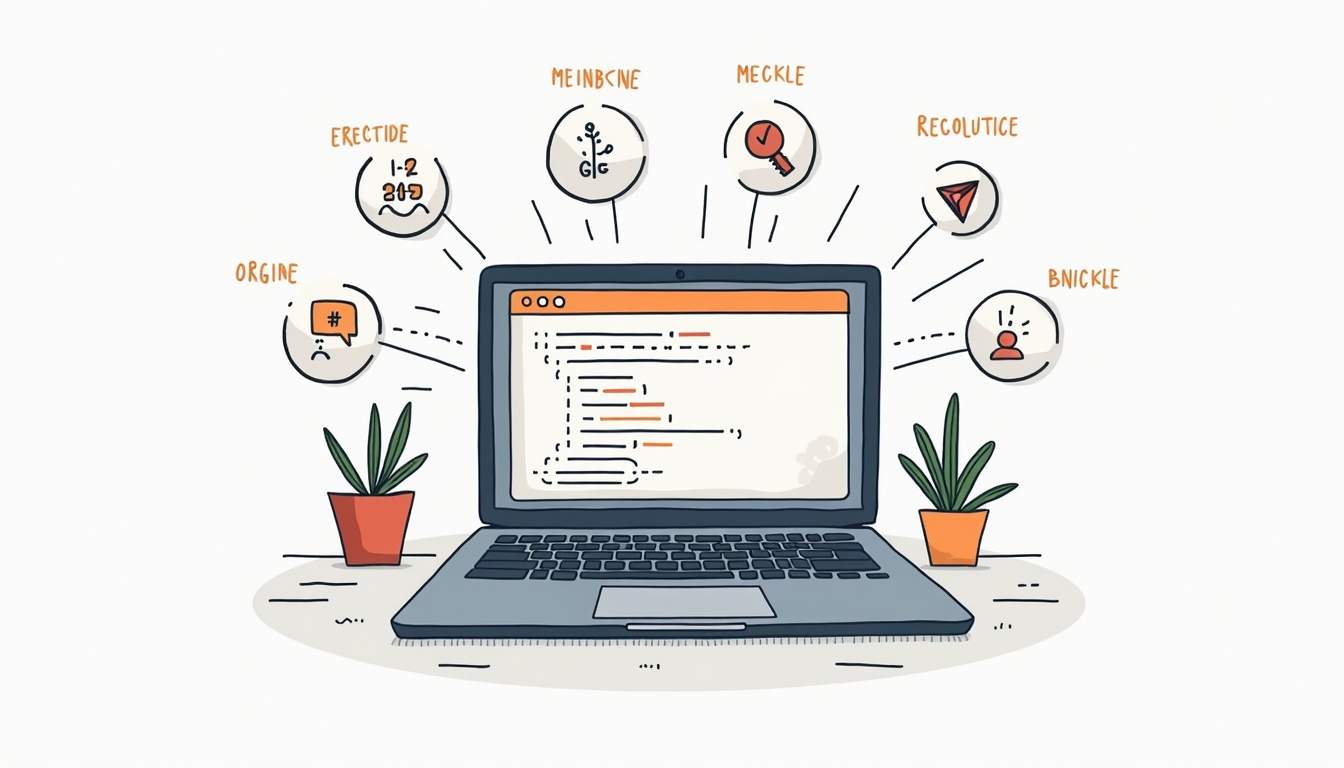
When to Delete a Branch
Once you have merged your feature or fix into the main branch and have confirmed that everything is working correctly, it's advisable to delete the corresponding branch. This practice keeps your repository clean and reduces clutter.
Keeping Your Git Repository Clean
Regularly review and delete branches that are no longer needed. This helps prevent confusion among team members and maintains a streamlined workflow. It’s also good practice to periodically perform cleanup operations to remove stale branches both locally and remotely.
By following these steps, you'll make the process of deleting branches in Git straightforward and efficient. Remember that effective management of branches is key to smoother collaborations and project workflows in Git.
Streamline Your Development Workflow with Engine Labs
Now that you understand the importance of keeping your Git repository clean and the best practices for deleting branches, take your team's efficiency to the next level with Engine Labs. Engine is not just about maintaining a tidy codebase; it's about revolutionizing your entire development process. By integrating with your favorite project management tools, Engine Labs automates up to half of your tickets, turning them into pull requests with incredible speed. Say goodbye to the backlog and hello to faster shipping with Engine. Ready to embrace the future of software engineering? Get Started with Engine Labs today and watch your projects soar.